How to Export SQL Data in CSV with a DATE Filter in PHP
Learn how to export SQL data in CSV with a date filter using PHP. Discover step-by-step instructions and code examples to efficiently retrieve and export specific data from your SQL database based on a desired date range.
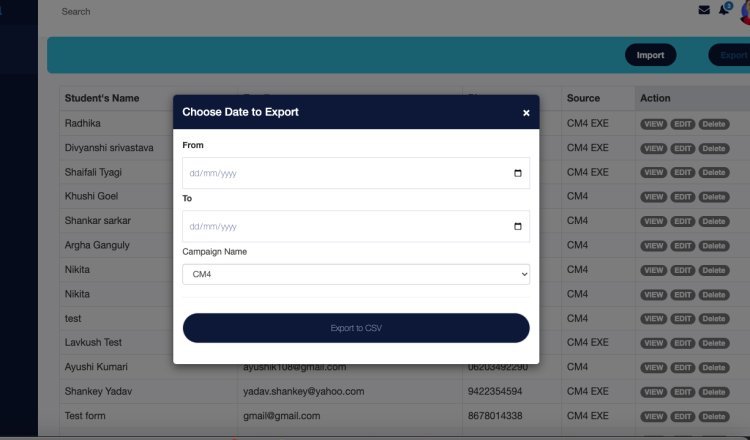
CSV stands for Comma Separated Value. It is well known file format to store tabular data or excel data. In this post you will learn how to export data to csv file with date range filter using PHP and MySQL.
To export SQL data with a date filter in PHP, you can follow these steps:
Step - 1:
Establish a connection to your database using PHP's mysqli
or PDO
extension. Make sure you have the necessary credentials and server information.
Step - 2:
Construct your SQL query: Build a SQL query that includes the desired date filter. For example, if you want to export data from a specific table (your_table
) with a date column (date_column
), you can use the following query as a template:
Step - 3:
$startDate = '2023-01-01';
$endDate = '2023-12-31';
$query = "SELECT * FROM your_table WHERE date_column BETWEEN '$startDate' AND '$endDate'";
This query selects all the rows from your_table
where the date_column
falls within the specified date range.
Step - 4:
Execute the query: Use the appropriate functions (mysqli_query()
or PDO::query()
) to execute the SQL query.
Step - 5:
Fetch the data: Retrieve the data from the executed query using functions like mysqli_fetch_assoc()
or PDOStatement::fetch()
. You can iterate over the results to process or export the data as needed.
Step - 6:
Export the data: Depending on your requirements, you can export the fetched data to various formats such as CSV, Excel, or JSON. For example, to export as a CSV file, you can use the following code:
$filename = 'export.csv';
$delimiter = ','; // Specify your desired delimiter
$file = fopen($filename, 'w');
// Write the column headers
$columnHeaders = array('Column1', 'Column2', 'Column3'); // Replace with actual column names
fputcsv($file, $columnHeaders, $delimiter);
// Write the data rows
while ($row = mysqli_fetch_assoc($result)) {
fputcsv($file, $row, $delimiter);
}
fclose($file);
This code creates a CSV file named export.csv
and writes the fetched data into it, using the specified delimiter.
Step - 7:
Remember to handle errors, sanitize user input, and ensure the appropriate security measures (such as prepared statements or parameter binding) are implemented to protect against SQL injection attacks.
By following these steps, you can export SQL data with a date filter using PHP. But if you've stil any doubt then you can ask it in the comment section I'll reply you.
Thank You!